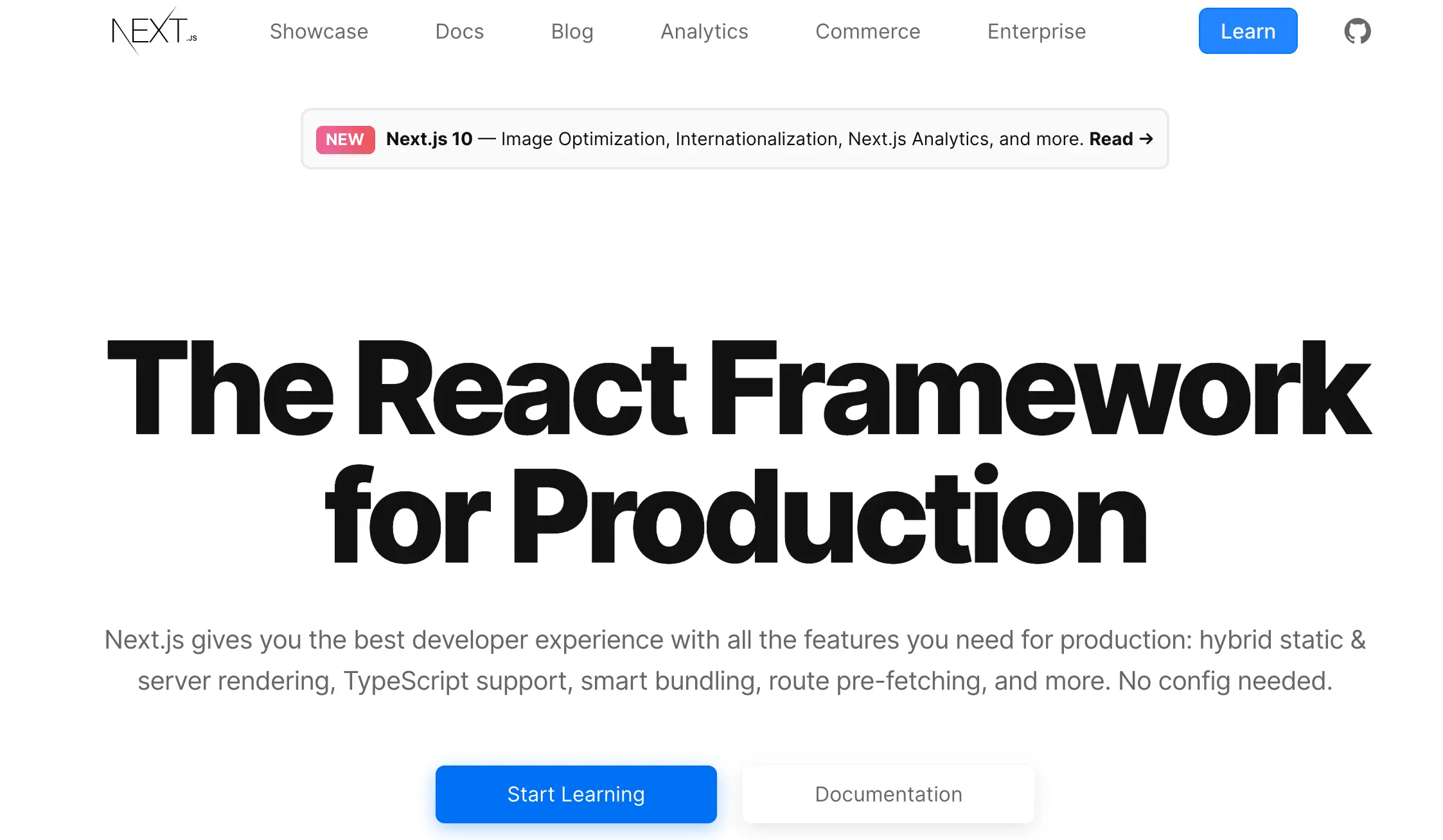
Advanced CSS Techniques for Modern Web Development
Modern CSS has evolved significantly, offering powerful features that make complex layouts and animations easier to implement. Let's explore some advanced techniques that will enhance your web development workflow.
CSS Grid Layout
1. Basic Grid Setup
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 20px;
padding: 20px;
}
2. Advanced Grid Patterns
.advanced-grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
grid-auto-rows: minmax(100px, auto);
grid-gap: 20px;
}
.grid-item {
grid-column: span 2;
grid-row: span 2;
}
Flexbox Mastery
1. Responsive Navigation
.nav {
display: flex;
justify-content: space-between;
align-items: center;
flex-wrap: wrap;
}
.nav-item {
flex: 1;
min-width: 100px;
text-align: center;
}
2. Card Layout
.card-container {
display: flex;
flex-wrap: wrap;
gap: 20px;
}
.card {
flex: 1 1 300px;
display: flex;
flex-direction: column;
}
CSS Custom Properties
1. Theme Variables
:root {
--primary-color: #007bff;
--secondary-color: #6c757d;
--spacing-unit: 8px;
--border-radius: 4px;
}
.button {
background-color: var(--primary-color);
padding: calc(var(--spacing-unit) * 2);
border-radius: var(--border-radius);
}
2. Dynamic Theming
[data-theme="dark"] {
--bg-color: #1a1a1a;
--text-color: #ffffff;
--accent-color: #00ff00;
}
[data-theme="light"] {
--bg-color: #ffffff;
--text-color: #1a1a1a;
--accent-color: #007bff;
}
Modern Layout Patterns
1. Holy Grail Layout
.holy-grail {
display: grid;
grid-template-areas:
"header header header"
"nav main aside"
"footer footer footer";
grid-template-columns: 200px 1fr 200px;
grid-template-rows: auto 1fr auto;
min-height: 100vh;
}
.header { grid-area: header; }
.nav { grid-area: nav; }
.main { grid-area: main; }
.aside { grid-area: aside; }
.footer { grid-area: footer; }
2. Masonry Layout
.masonry {
columns: 3;
column-gap: 20px;
}
.masonry-item {
break-inside: avoid;
margin-bottom: 20px;
}
Advanced Animations
1. Keyframe Animations
@keyframes slideIn {
from {
transform: translateX(-100%);
opacity: 0;
}
to {
transform: translateX(0);
opacity: 1;
}
}
.animate {
animation: slideIn 0.5s ease-out forwards;
}
2. Scroll-Triggered Animations
.scroll-animate {
opacity: 0;
transform: translateY(20px);
transition: all 0.6s ease-out;
}
.scroll-animate.visible {
opacity: 1;
transform: translateY(0);
}
Modern CSS Features
1. Container Queries
@container (min-width: 400px) {
.card {
display: grid;
grid-template-columns: 200px 1fr;
}
}
2. CSS Scroll Snap
.scroll-container {
scroll-snap-type: x mandatory;
overflow-x: scroll;
display: flex;
}
.scroll-item {
scroll-snap-align: start;
flex: 0 0 100%;
}
Performance Optimization
1. Will-change Property
.optimized {
will-change: transform;
transform: translateZ(0);
}
2. Content-visibility
.lazy-content {
content-visibility: auto;
contain-intrinsic-size: 0 500px;
}
Best Practices
- Use CSS Custom Properties for maintainable theming
- Implement responsive designs using modern layout techniques
- Optimize animations for performance
- Use CSS Grid and Flexbox appropriately
- Implement progressive enhancement
- Consider browser support and fallbacks
Conclusion
Modern CSS provides powerful tools for creating sophisticated layouts and animations. By mastering these advanced techniques, you can create more maintainable, performant, and visually appealing web applications. Remember to consider browser support and implement appropriate fallbacks for older browsers.