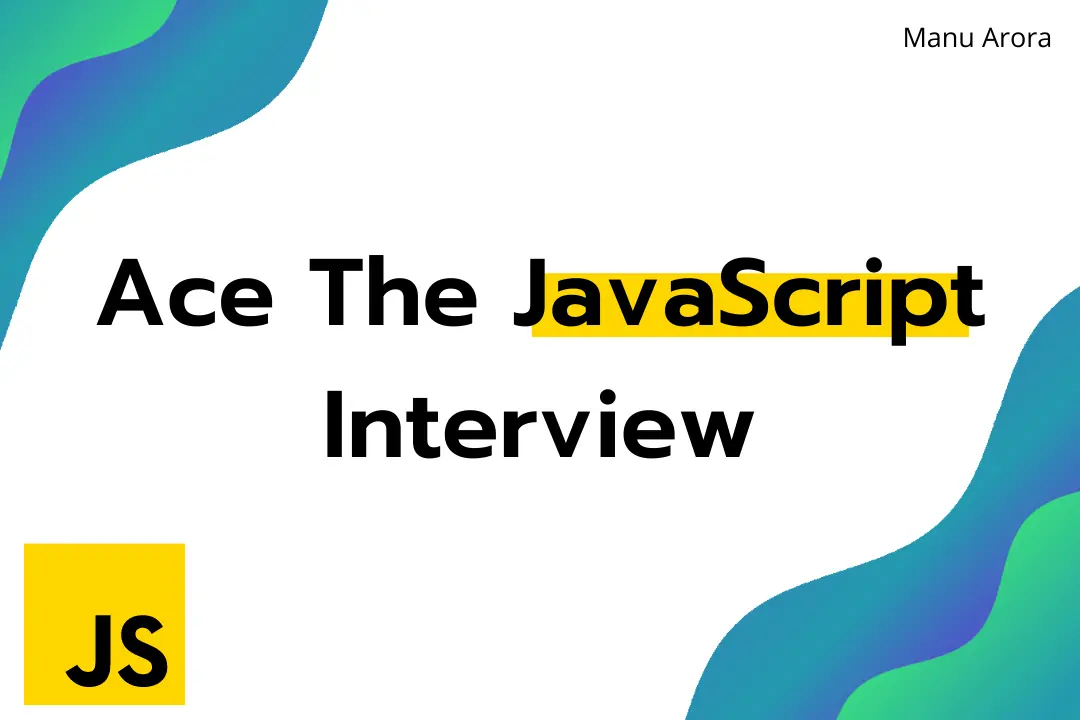
Web Performance Optimization: A Comprehensive Guide
Web performance optimization is crucial for providing a better user experience and improving your site's SEO ranking. Let's explore various techniques and best practices to make your web applications faster and more efficient.
Core Web Vitals
1. Largest Contentful Paint (LCP)
// Monitor LCP
new PerformanceObserver((entryList) => {
for (const entry of entryList.getEntries()) {
console.log('LCP:', entry.startTime);
}
}).observe({ entryTypes: ['largest-contentful-paint'] });
2. First Input Delay (FID)
// Monitor FID
new PerformanceObserver((entryList) => {
for (const entry of entryList.getEntries()) {
console.log('FID:', entry.processingStart - entry.startTime);
}
}).observe({ entryTypes: ['first-input'] });
Code Optimization
1. Code Splitting
// React example with dynamic imports
const MyComponent = React.lazy(() => import('./MyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<MyComponent />
</Suspense>
);
}
2. Tree Shaking
// webpack.config.js
module.exports = {
mode: 'production',
optimization: {
usedExports: true,
sideEffects: true
}
};
Image Optimization
1. Responsive Images
<picture>
<source
srcset="image-small.webp 300w,
image-medium.webp 600w,
image-large.webp 900w"
sizes="(max-width: 600px) 300px,
(max-width: 900px) 600px,
900px"
type="image/webp">
<img src="fallback.jpg" alt="Description">
</picture>
2. Lazy Loading
<img
src="image.jpg"
loading="lazy"
alt="Description"
width="800"
height="600">
Caching Strategies
1. Service Worker
// service-worker.js
const CACHE_NAME = 'v1';
const urlsToCache = [
'/',
'/styles.css',
'/app.js'
];
self.addEventListener('install', event => {
event.waitUntil(
caches.open(CACHE_NAME)
.then(cache => cache.addAll(urlsToCache))
);
});
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request)
.then(response => response || fetch(event.request))
);
});
2. HTTP Caching Headers
# nginx.conf
location /static/ {
expires 1y;
add_header Cache-Control "public, no-transform";
}
Resource Optimization
1. Minification
// webpack.config.js
const TerserPlugin = require('terser-webpack-plugin');
module.exports = {
optimization: {
minimize: true,
minimizer: [new TerserPlugin()],
},
};
2. Compression
// Express.js example
const compression = require('compression');
app.use(compression());
Performance Monitoring
1. Real User Monitoring (RUM)
// Basic RUM implementation
window.addEventListener('load', () => {
const timing = window.performance.timing;
const metrics = {
dns: timing.domainLookupEnd - timing.domainLookupStart,
tcp: timing.connectEnd - timing.connectStart,
request: timing.responseEnd - timing.requestStart,
dom: timing.domComplete - timing.domLoading,
load: timing.loadEventEnd - timing.navigationStart
};
// Send metrics to analytics
sendToAnalytics(metrics);
});
2. Performance Budgets
// webpack.config.js
module.exports = {
performance: {
maxAssetSize: 244000,
maxEntrypointSize: 244000,
hints: 'error'
}
};
Best Practices
- Implement code splitting and lazy loading
- Optimize and compress images
- Use appropriate caching strategies
- Minimize and compress assets
- Monitor performance metrics
- Implement progressive enhancement
- Use modern image formats (WebP, AVIF)
- Optimize third-party scripts
- Implement critical CSS
- Use resource hints (preload, prefetch)
Tools and Resources
1. Performance Testing Tools
- Lighthouse
- WebPageTest
- Chrome DevTools Performance panel
- GTmetrix
2. Optimization Tools
- ImageOptim
- Webpack Bundle Analyzer
- Compression plugins
- Minification tools
Conclusion
Web performance optimization is an ongoing process that requires attention to detail and regular monitoring. By implementing these techniques and best practices, you can significantly improve your website's performance and provide a better user experience. Remember to measure and monitor your performance metrics regularly to ensure your optimizations are effective.